반응형
SMALL
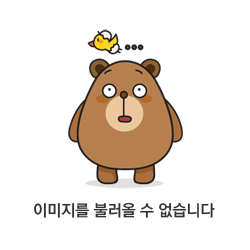
[1] 함수
(1) 함수 정의 funcion
함수를 호출하여 수행하고 , 수행이 끝나면 반환된다
함수로 구현된 기능은 여러 곳에서 호출되어 사용이 가능하다
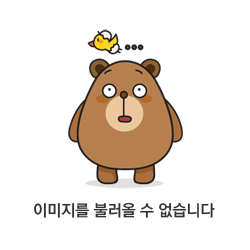
(2) 함수의 구성
함수 이름 : add
매개 변수 : num1 , num2
반환 값 : return sum
몸체 : { } 전부
int add (int num1, int num2) { // num1 과 num2가 주어졌을때
int sum;
sum = num1 + num2;
return sum;
} // add함수의 반환값은 sum이다
(3) 클래스 안에서 함수 사용하는 방법
public class Test {
// 1. addSum 함수
public static int addSum(int num1, int num2) {
int sum;
sum = num1 + num2;
return sum;
}
// 2. sayHello 함수
public static void sayHello(String greeting) {
System.out.println(greeting);
}
// 3. calcSum 함수
public static int calcSum() {
int sum = 0;
for(int i = 0; i<=100; i++) {
sum += i;
}
return sum;
}
// 4. 본 main 함수
public static void main(String[] args) {
int n1 = 10;
int n2 = 20;
int total = addNum(n1, n2); // 1번 함수 사용
sayHello("안녕하세요"); // 2번 함수 사용
int num = calcSum(); // 3번 함수 사용
System.out.println(total);
System.out.println(num);
}
[2] 메서드
(1) 메서드 정의
- 객체의 기능을 구현하기 위해 클래스 내부에 구현되는 함수
- 메서드를 구현하여 객체의 기능이 구현된다
- getStudentName()
- 멤버 변수를 이용하여 클래스의 기능을 구현한 함수
- 작업을 수행하는데 필요한 값만 넣고 원하는 결과를 얻으면 되고 어떤 과정을 거치는지는 몰라도 된다
(2) 메서드 구성
MyMath mm = new MyMath(); //인스턴스
long num = mm.add(1L,2L); // 인스턴스에 메서드 적용
long add(long a, long b){ //메서드
long result = a+b;
return result;
}
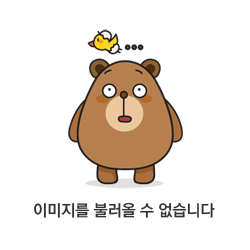
- 선언부(메서드 이름 / 매개변수 선언 / 반환타입) + 구현부
- 구현부는 반환값이 반드시 포함되어있어야한다
(3) return 반환값
(3-1) 반환타입 void (O) return 생략
반환타입이 void 일경우 return 단어를 생략해도된다. 컴파일시 자동으로 추가가 된다
void test(int num) {
.......
System.out.println(..);
}
(3-2) 반환타입 void (O) return 필수
반환타입이 void가 아니므로 반드시 return이 있어야한다
int test(int num) {
.......
return result;
}
(3-3) 조건문일때 return 필수
조건의 true , false에 따라 각각 return 은 필수이다
int max (int a, int b) {
if( a>b) return a;
else return b
}
(3-4) return 값은 변수 또는 수식 가능
int test(int num) {
.......
return result;
}
int test(int a, int b) {
.......
return a+b;
}
(3-5) return 반환타입이 참조형이 가능
모든 참조형 타입의 값은 객체의 주소이다
public static void main(String[] args) {
Student studentA = new Student;
}
static Student copy(Student studentA) (
Student test = new Student();
return test;
}
(4) 메서드의 매개변수
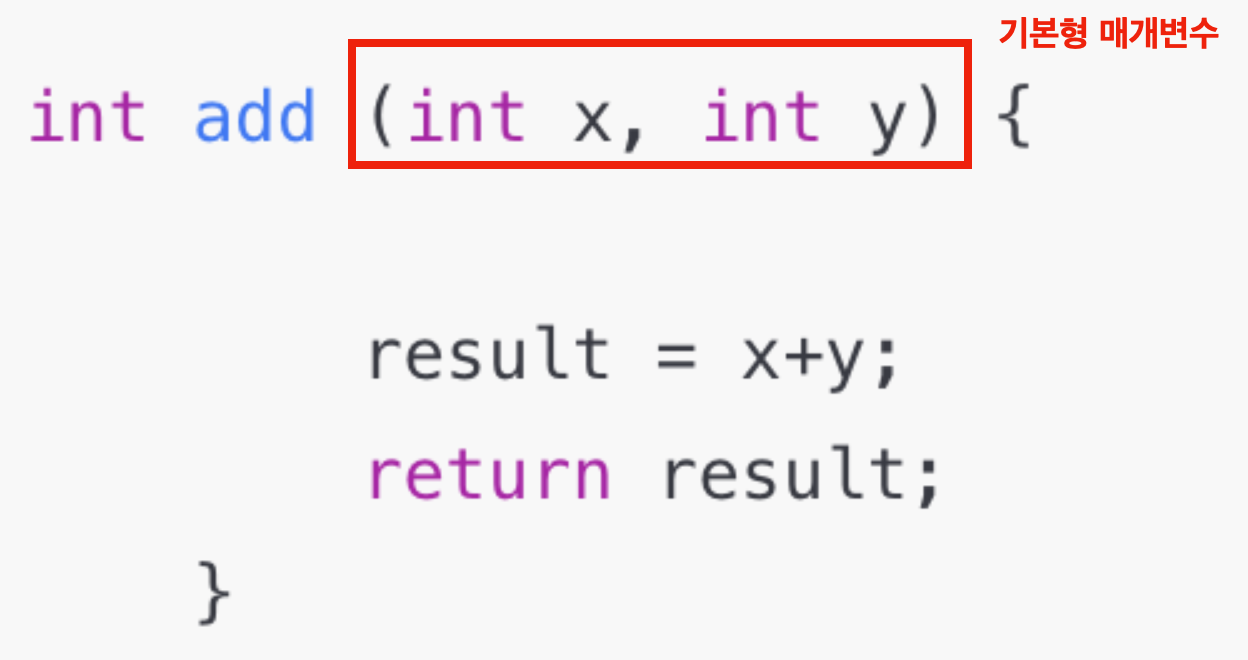
기본형 매개변수 : 기본형 값이 복사되어 변수값을 읽기만 한다
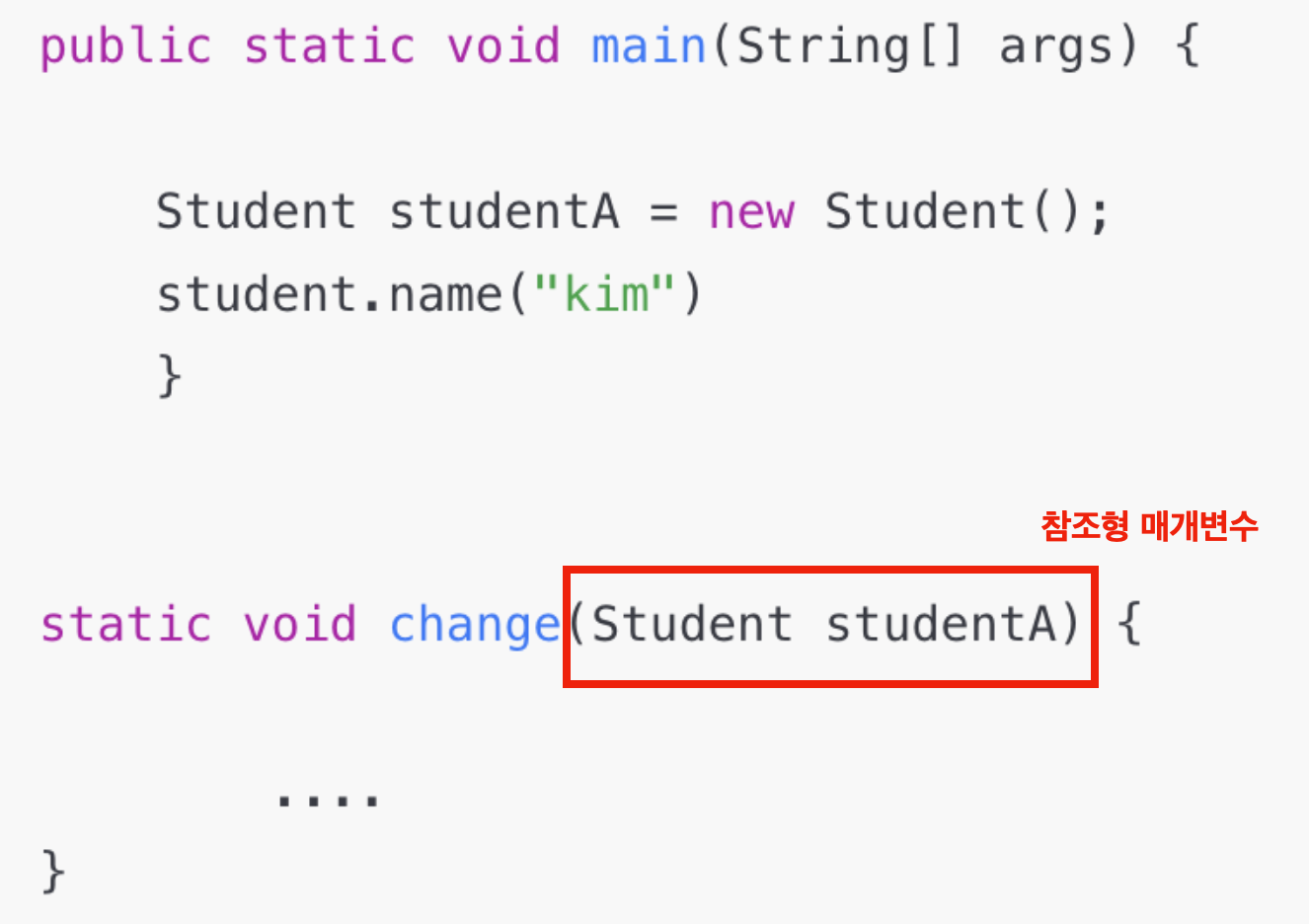
참조형 매개변수 : 인스턴스의 주소가 복사되어 변수값을 읽고 변경이 가능하다
(5) 호출스택 call stack
메서드가 호출되면 수행에 필요한 만큼의 메모리를 스택에 할당받는다
메서드가 수행을 마치고 나면 사용했던 메모리를 반환하고 스택에서 제거된다
호출 스택의 제일 위에 있는 메서드가 현재 실행 중인 메서드이다
아래에 있는 메서드가 바로 위의 메서드를 호추한 메서드이다
- 스택에 함수가 호출될때 지역변수들이 메모리 공간을 차지하고 함수 사용이 종료되면 메모리가 반환된다
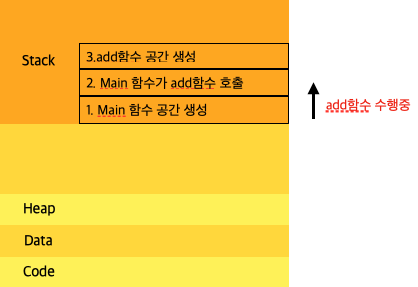
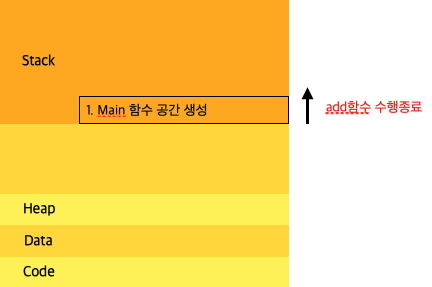
(6) 인스턴스 메서드 & static 메서드
인스턴스 메서드 : 인스턴스 변수 , static 변수 둘다 ok
static 메서드 : static 변수만 ok
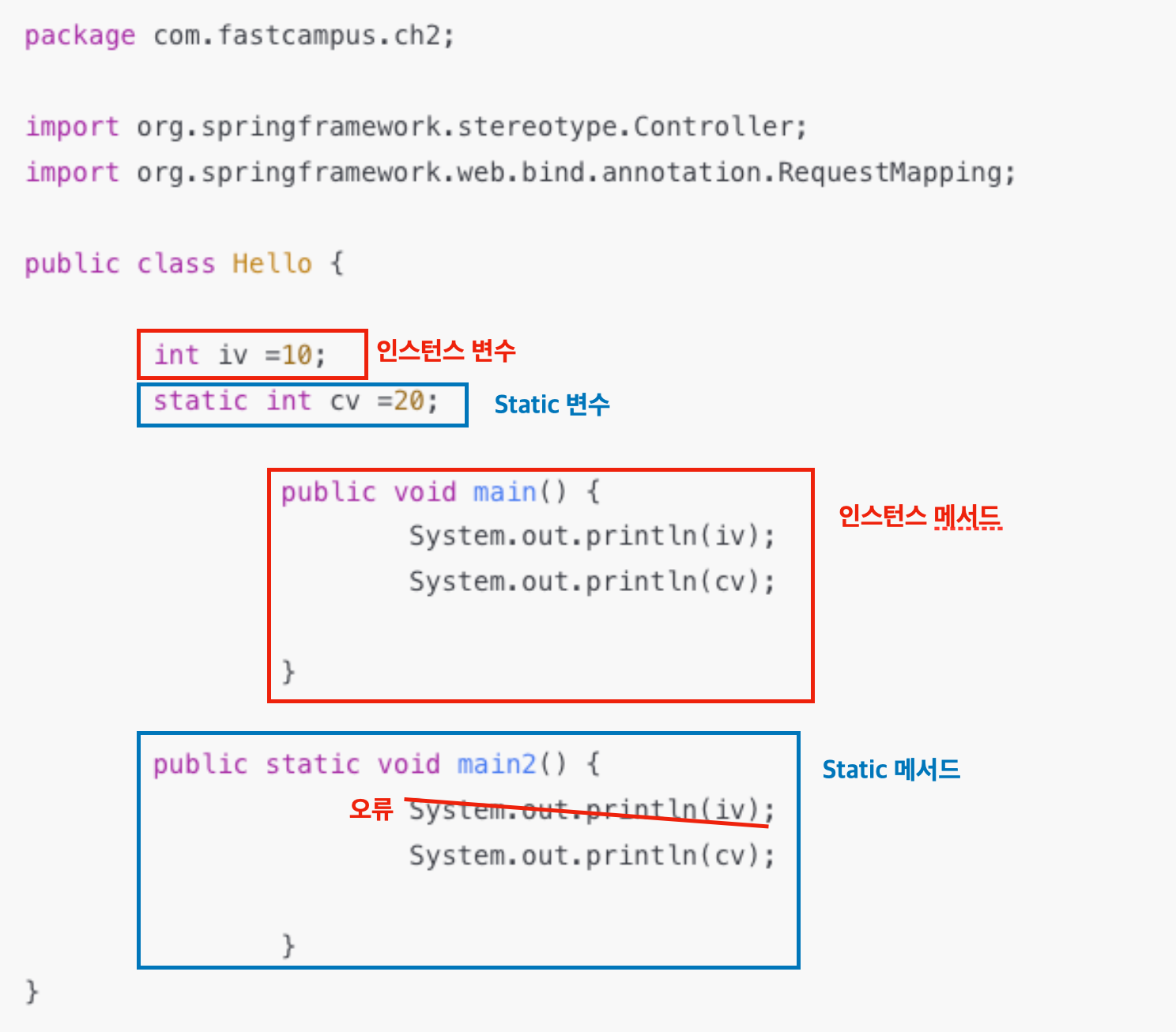
(2) 클래스 안에 멤버변수와 메서드를 구현한다
- 학생이라는 클래스에 멤버변수 ( 학번, 학생이름, 주소) 와 메서드 (학생의 정보 조회 , 학생이름 가져오기) 를 만든다
public class Student {
// 멤버변수
public int studentID;
public String studentName;
public String address;
// 메서드 = 멤버함수
public void showStudentInfo() {
System.out.println(studentName + "," + address);
}
public String getStudentName() {
return studentName;
}
}
- 본 main 클래스에서 학생클래스 정보를 호출하여 수행한다
public class Service {
public static void main(String[] args) {
//인스턴스1
Student studentA = new Student();
studentA.studentName = "김철수";
studentA.address = "서울";
studentA.showStudentInfo(); // 메서드 호출
//인스턴스2
Student studentB = new Student();
studentB.studentName = "이영희";
studentB.address = "경기도";
studentB.showStudentInfo(); //메서드 호출
System.out.println(studentA);
System.out.println(studentB);
}
}
[3] 참조 자료형
(1) 참조 자료형
- String 문자열 , Student 변수 등
- 메모리크기가 int, long 처럼 지정되어있지 않아 별도로 클래스로 만들어서 호출해야한다
- 클래스를 만들어 변수를 선언해야한다
- 참조 자료형 변수를 호출할때는 변수를 new 생성해야한다
(2) 참조자료형 , 함수 , 인스턴스 사용하는 예시
- (클래스 Student) 멤버변수, 함수 ,메서드 정의한다
package 패키지;
public class Student {
//멤버변수
int studentID;
String studentName;
//참조자료형 변수
Subject korea;
Subject math;
//생성자 정의
public Student(int id, String name) {
studentID = id;
studentName = name;
//참조자료형 변수 호출하기
korea = new Subject();
math = new Subject();
}
//메서드 생성
public void setKoreaSubject(String name, int score) {
korea.subjectName = name;
korea.score = score;
}
//메서드 생성
public void setMathSubject(String name, int score) {
math.subjectName = name;
math.score = score;
}
//메서드 생성
public void showStudentSocre() {
int total = korea.score + math.score;
System.out.println(studentName + " 학생의 총점은 " + total + "점 입니다." );
}
}
- 참조자료형의 클래스
package 패키지;
public class Subject {
//멤버변수
String subjectName;
int score;
int subjectID;
}
- 본 main에서 인스턴스 생성. 인스턴스에 정보 넣기 , 메서드 호출
package 패키지;
public class Service {
public static void main(String[] args) {
//인스턴스1
Student studentA = new Student(100, "Lee");
//인스턴스1에 정보넣기
studentA.setKoreaSubject("국어", 100);
studentA.setMathSubject("수학", 95);
//인스턴스2
Student studentB = new Student(101, "Kim");
studentB.setKoreaSubject("국어", 80);
studentB.setMathSubject("수학", 99);
//메서드 호출
studentA.showStudentSocre();
studentB.showStudentSocre();
}
}
반응형
LIST
'🌈 백엔드 > 객체 지향' 카테고리의 다른 글
JAVA_객체지향_생성자 (0) | 2023.06.03 |
---|---|
JAVA_객체지향_인스턴스 & static 클래스변수 (0) | 2023.06.03 |
JAVA_객체지향_프로그래밍 (0) | 2023.06.03 |
JAVA_객체지향_ 예외 클래스 (0) | 2023.02.16 |
JAVA_객체지향_객체 & 클래스 (0) | 2023.02.15 |