[1] 배열
(1) 배열 정의
- 순차적 자료구조 = 선형 자료구조
- 동일한 데이터 타입을 순서에 따라 관리하는 자료 구조
- 같은 타입의 여러 변수를 하나의 묶음으로 다루는 것
중복값이 없다는 전제라면 자료구조는 집합을 고려하고 아니라면 자료구조는 배열로 선택해야한다
(2) 배열 특징
- 물리적 위치와 논리적 위치가 동일하다
- 인덱스 연산자를 이용하여 참조한다
- 배열의 순서는 0부터 시작한다
- 정해진 크기가 있음
- 배열의 요소를 추가하거나 삭제하면 다른 요소들의 이동에 대해 구현하는 작업을 해야한다
- 요소의 개수가 배열의 길이보다 커지만 배열을 재할당하고 복사하는 작업이 필요하다
- 각 저장공간이 연속적으로 배치되어있다
(3) 배열의 길이
- 배열의 크기는 정해져있다
- 메모리 공간안에 배열이 저장된다 .
- 메모리 공간이 배열의 크기만큼 공간이 없다면 배열을 저장할수 없다
- 배열의 크기로 인해 메모리 공간을 효율적으로 사용하기 어렵다
- 배열을 선언하면 배열의 크기만큼 메모리가 할당되지만, 실제 요소(데이터)가 없을수 있다
- 배열의 length 속성은 배열의 개수, 크기를 반환해주기 때문에 실제 요소의 개수와는 다르다
- 자바가상머신 JVM이 모든 배열의 길이를 별도로 관리한다
배열의 크기와 상관없이 저장된 실제 값만 곱한 결과를 출력한다
//배열의 길이는 5이지만 실제값은 3개밖에 안들어있다. 실제 값만 곱한 결과를 얻으려면 count를 만들어서 움직인다
double[] arr = new double[5];
int count = 0;
arr[0] = 1.1; count++;
arr[1] = 2.1; count++;
arr[2] = 3.1; count++; //count =3
double total = 1;
for(int i = 0; i< count; i++) {
total *= arr[i];
}
System.out.println(total); // 실제값만 곱한 결과
배열의 크기 구하기
arr [] = {1,2,3,4,5}
for(int i=0; i < arr.length; i++) {
count++;
}
return count =5 // 배열의 크기는 5
(4) 배열 선언 및 초기화
boolean | false |
char | \u0000 |
byte short int | 0 |
long | 0L |
float | 0.0 |
double | 0.0 |
- 변수는 배열을 다루는데 필요한 참조변수이고 값이 저장되는 공간이 아니다
- 배열을 선언하는 것은 배열을 다루기 위해 참조변수의 공간을 만드는것이고 new int[10] 처럼 배열의 길이와 타입을 지정해야 배열이 생성된다 . 이때 실제 저장공간이 생성된다
- 배열은 생성과 동시에 기본값 0으로 초기화된다 . 하지만 값을 저장하려면 각 인덱스 마다 값을 배정해줘야한다
- {1,2,3} 이렇게 값을 넣어 초기화를 할경우 배열의 길이를 명시하지 않더라도 요소 갯수에 따라 자동으로 결정된다
int[] arr = new int[10];
int arr[] = new int[10];
int[] arr = new int[] {10, 20, 30};
int[] arr = {10, 20, 30};
int[] arr;
arr = new int[] {10, 20, 30};
arr [0] = 1
arr [1] = 2
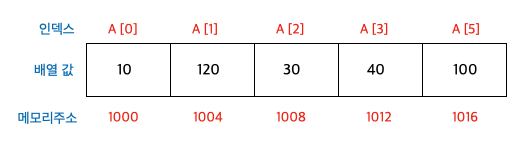
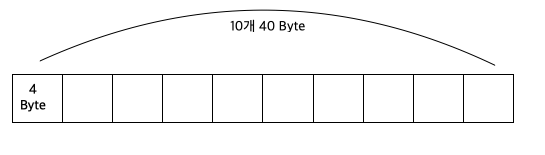
[2]객체 배열
(1) 객체 배열
- 객체를 요소로 가지고 있는 배열은 객체의 주소가 메모리에 할당된 것으로 , 각 요소는 객체를 생성 및 저장을 해야한다
- 객체 참조형의 초기화는 null
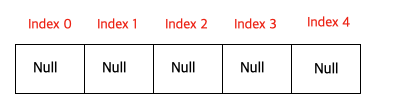
(2) 객체 배열 선언과 초기화
String [] arr = new String[5];
String[] arr= new String[] {kim,lee,min}
String[] arr ={kim,lee,min}
arr[0] = new String("kim")
arr[1] = new String("lee")
arr[0] ="kim"
arr[1] ="lee"
(3) 객체 배열을 만들기
- 클래스에 멤버변수 , 생성자 , 캡슐화 작업을 한다
public class Book {
//멤버변수
private String title;
private String author;
//생성자
public Book() {}
public Book(String title, String author) {
this.title = title;
this.author = author;
}
//캡슐화
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
// 메서드
public void showBookInfo() {
System.out.println(title + "," +author);
}
}
- 배열의 크기를 5로 설정하여 객체 배열을 만든다
- 각 요소의 값을 넣었을때 생성된 객체의 주소값이 배정된다
public class Service {
public static void main(String[] args) {
Book[] library = new Book[5]; // null 값만 들어가있음
//각 요소에 값을 넣는다
library[0] = new Book("책1", "작가1");
library[1] = new Book("책2", "작가2");
library[2] = new Book("책3", "작가3");
library[3] = new Book("책4", "작가4");
library[4] = new Book("책5", "작가5");
for(int i =0; i<library.length; i++) {
System.out.println(library[i]);
library[i].showBookInfo();
}
}
}
(3) 객체 배열을 복사하기 : 얕은 복사
- 객체는 변함없이 객체의 주소만 복사되어 기존 배열 또는 복사 배열 중에 한개라도 수정되면 같이 수정된다
public class ObjectCopy {
public static void main(String[] args) {
Book[] library = new Book[5];
Book[] copyLibaray = new Book[5];
library[0] = new Book("책1", "작가1");
library[1] = new Book("책2", "작가2");
library[2] = new Book("책3", "작가3");
library[3] = new Book("책4", "작가4");
library[4] = new Book("책5", "작가5");
// 얕은복사
System.arraycopy(library, 0, copyLibaray, 0, 5);
System.out.println("======copy library=========");
for( Book book : copyLibaray ) {
book.showBookInfo();
}
// 요소값 변경
library[0].setTitle("책변경");
library[0].setAuthor("작가변경");
System.out.println("======library=========");
for( Book book : library) {
book.showBookInfo();
}
System.out.println("======copy library=========");
for( Book book : copyLibaray) {
book.showBookInfo();
}
}
}
(4) 객체 배열을 복사하기 : 깊은 복사
- 복사할때 각각의 객체를 생성하여 객체의 값 자체를 복사하기 때문에 다른 객체를 가리킨다
public class ObjectCopy {
public static void main(String[] args) {
Book[] library = new Book[5];
Book[] copyLibaray = new Book[5];
library[0] = new Book("책1", "작가1");
library[1] = new Book("책2", "작가2");
library[2] = new Book("책3", "작가3");
library[3] = new Book("책4", "작가4");
library[4] = new Book("책5", "작가5");
// 깊은 복사
copyLibaray[0] = new Book();
copyLibaray[1] = new Book();
copyLibaray[2] = new Book();
copyLibaray[3] = new Book();
copyLibaray[4] = new Book();
for(int i = 0; i< library.length; i++) {
copyLibaray[i].setTitle(library[i].getTitle());
copyLibaray[i].setAuthor(library[i].getAuthor());
}
library[0].setTitle("변경책");
library[0].setAuthor("변경작가");
System.out.println("======library=========");
for( Book book : library) {
book.showBookInfo();
}
System.out.println("======copy library=========");
for( Book book : copyLibaray) {
book.showBookInfo();
}
}
}
[3] 다차원배열
(1) 다차원배열
- 2차원 이상으로 구현된 배열
(2) 다차원 배열 선언과 초기화
int [][] arr ;
int arr [][];
int[] arr[];
int [][] arr = new int [2][3]
int [][] arr = new int [][] { {1,2,3} , {4,5,6} }
int [][] arr = {{1,2,3}, {4,5,6}}
int [][] arr = {
{1,2,3},
{4,5,6}
}
arr[0][0] = 100;
System.out.println(arr[0][0]);
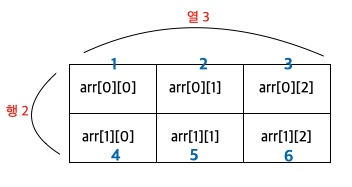
int A [3][2] = [행][열]
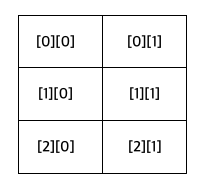
(3) 2차원 배열 예시
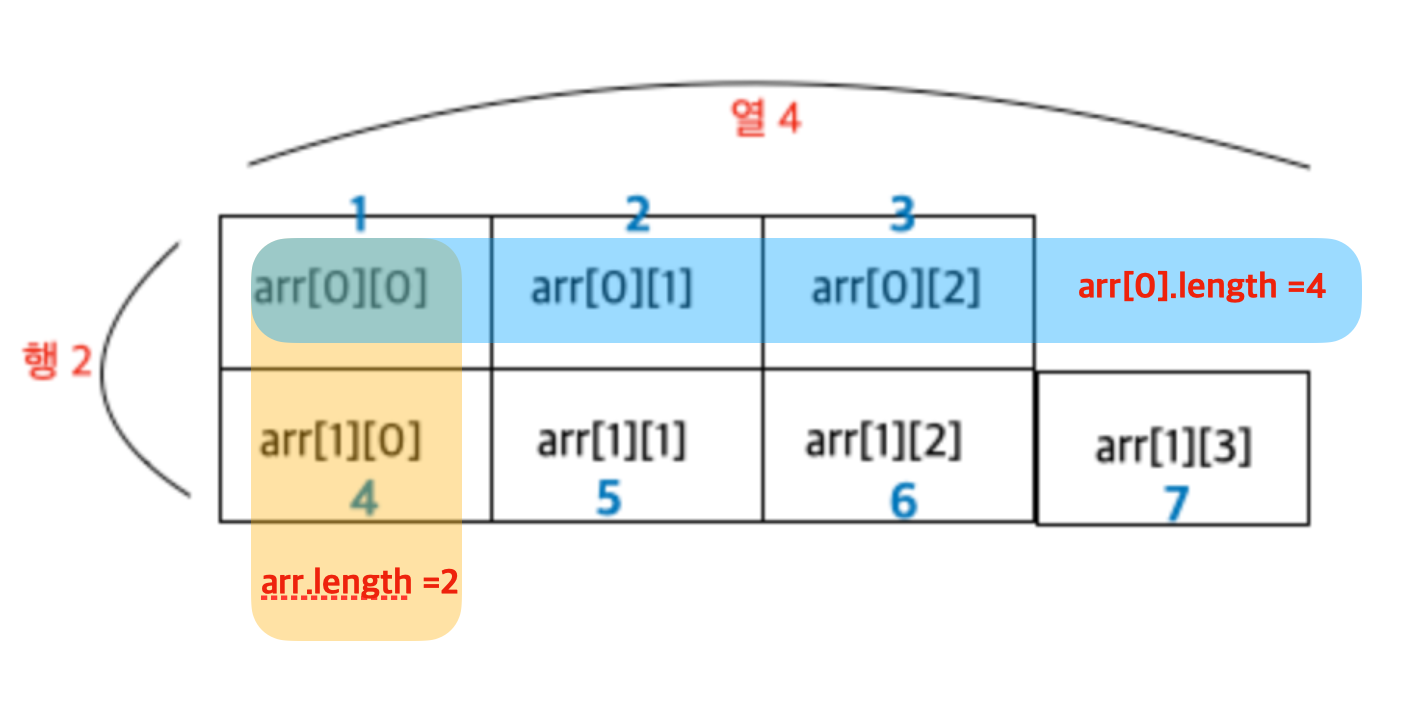
public class Test {
public static void main(String[] args) {
int[][] arr = {
{1,2,3},
{4,5,6,7}
};
for(int i = 0; i<arr.length; i++) {
for(int j=0; j<arr[i].length; j++) {
System.out.print(arr[i][j] + " ");
}
System.out.println(", \t" + arr[i].length);
}
}
}
(4) 2차원 배열 예시
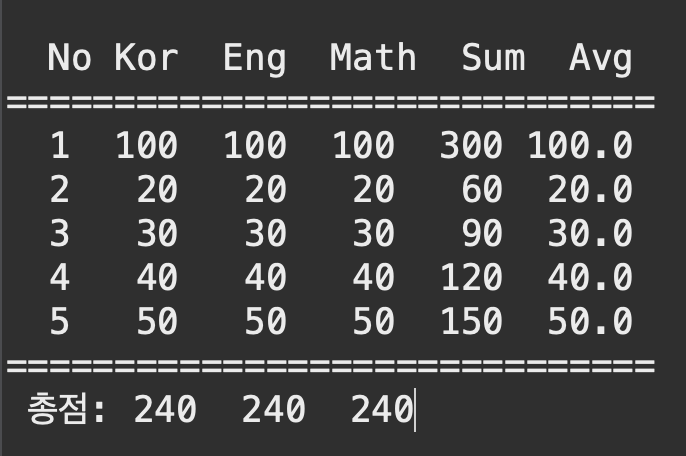
import java.util.Arrays;
public class HelloJava {
public static void main(String[] args) {
int [][] score ={
{100,100,100},
{20,20,20},
{30,30,30},
{40,40,40},
{50,50,50}
};
System.out.println(" No Kor Eng Math Sum Avg");
System.out.println("==============================");
int korSum =0;
int engSum =0;
int mathSum =0;
for(int i=0; i<score.length;i++) {
korSum +=score[i][0];
engSum +=score[i][0];
mathSum +=score[i][0];
System.out.printf("%3d",i+1);
int sum =0;
float avg =0.0f;
for(int j=0; j <score[i].length;j++) {
sum += score[i][j];
System.out.printf("%5d", score[i][j]);
}
avg = sum/ (float)score[i].length;
System.out.printf("%5d %5.1f%n",sum,avg);
}
System.out.println("==============================");
System.out.printf("총점:%4d %4d %4d", korSum,engSum,mathSum);
}
}
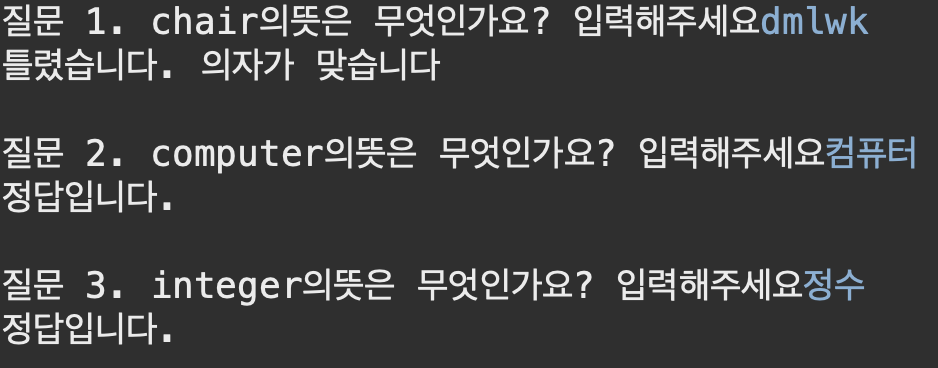
import java.util.Arrays;
import java.util.Scanner;
public class HelloJava {
public static void main(String[] args) {
String[][] words ={
{"chair","의자"}, // 00 01
{"computer","컴퓨터"}, // 10 11
{"integer","정수"} // 20 21
};
Scanner scanner = new Scanner(System.in);
for(int i=0; i <words.length;i++) {
System.out.printf("질문 %d. %s의뜻은 무엇인가요? 입력해주세요", i+1,words[i][0]);
String tmp =scanner.nextLine();
if(tmp.equals(words[i][1])) {
System.out.printf("정답입니다.%n%n");
}else {
System.out.printf("틀렸습니다. %s가 맞습니다%n%n",words[i][1]);
}
}
}
}
(5) 3차원배열
int A [2][3][2]

[4] 시간복잡도
(1) 읽기 : O(1)
arr[] = { 김, 신, 이 , 박, 원 , 윤}
arr [0] | arr[1] | arr[2] | arr[3] | arr[4] | arr[5] | |
값 | 김 | 신 | 이 | 박 | 원 | 윤 |
인덱스 | 0 | 1 | 2 | 3 | 4 | 5 |
메모리 주소 | 1010 | 1011 | 1012 | 1013 | 1014 | 1015 |
컴퓨터는 배열의 인덱스0 의 메모리주소를 기억하고 있기때문에 한번에 접근해서 읽을수 있다
하지만 컴퓨터는 인덱스3의 메모리주소를 모르기 때문에 값을 읽을 때는
인덱스0 의 메모리 주소를 불러온뒤 +3 을해서 인덱스 3의 메모리 주소를 찾고 값을 불러온다
+1,+2,+,3...이렇게 숫자를 더해서 값을 찾을수 있는 이유는 배열이 순차적으로 만들어졌기 때문이다
만약에 요소가 100개가 있는 배열에 어떤 요소를 읽는다면
연산에 걸리는 단계수 : 1번
(2) 검색 : O(N)
arr[] = { ?, ?, ?, ?, ?}
배열에서 "박" 이라는 값을 가지고 있는지 찾을 거다
arr [0] | arr[1] | arr[2] | arr[3] | arr[4] | arr[5] | |
값 | ? | ? | ? | ? | ? | ? |
인덱스 | 0 | 1 | 2 | 3 | 4 | 5 |
메모리 주소 | 1010 | 1011 | 1012 | 1013 | 1014 | 1015 |
그런데 인덱스0~5까지 "박"이라는 값을 가진 인덱스가 몇번인지 모르기 때문에 한개씩 들춰서 찾는다
인덱스 0 에 "박" 있니? 없어
인덱스 1 에 "박" 있니? 없어
인덱스 2 에 "박" 있니? 없어
인덱스 3 에 "박" 있니? 있어 , 찾았다
연산이 4번 걸렸다
선형검색 = 컴퓨터가 한 인덱스씩 검색하는 것
이러한 경우가 선형검색이다.
선형검색은 찾는 값이 나올때까지 순서대로 검색하기 때문에
운이 좋아 값이 인덱스 앞쪽에 있으면 빨리 찾을수 있지만
맨뒤에 있으면 값을 찾는데까지 연산도 오래걸리고 시간도 오래 걸린다
만약에 요소가 100개가 있는 배열에 어떤 요소를 검색한다면
연산에 걸리는 단계수 : 100번
(3) 삽입 : O(2N+1)
arr[] = { 김, 신, 이 , 박, 원 , 윤}
arr [0] | arr[1] | arr[2] | arr[3] | arr[4] | arr[5] | |
값 | 김 | 신 | 이 | 박 | 원 | 윤 |
인덱스 | 0 | 1 | 2 | 3 | 4 | 5 |
메모리 주소 | 1010 | 1011 | 1012 | 1013 | 1014 | 1015 |
(상황 1) 마지막 인덱스에 "민"을 넣는다
컴퓨터는 메모리 주소 1015 다음 번호인 1016에 "민"을 값으로 추가하면된다
(상황 2) 처음이나 중간에 있는 인덱스2 위치에 "민"을 추가한다
넣을수 있는 메모리 주소 공간이 없기 때문에
이, 박, 원, 윤 값을 한칸씩 뒤로 밀어야한다
"윤" 을 인덱스 6으로 바꾸고 , 메모리 주소도 1016으로 바꾼다
"원" 을 인덱스 5으로 바꾸고 , 메모리 주소도 1015으로 바꾼다
"박" 을 인덱스 4으로 바꾸고 , 메모리 주소도 1014으로 바꾼다
"이" 을 인덱스 3으로 바꾸고 , 메모리 주소도 1013으로 바꾼다
arr [0] | arr[1] | arr[2] | arr[3] | arr[4] | arr[5] | arr[6] | |
값 | 김 | 신 | 이 | 박 | 원 | 윤 | |
인덱스 | 0 | 1 | 2 | 3 | 4 | 5 | 6 |
메모리 주소 | 1010 | 1011 | 1012 | 1013 | 1014 | 1015 | 1016 |
그럼 인덱스 2의 값이 비어있는 상태이다
이때 "민" 을 인덱스 2에 추가한다
연산이 5번 발생했다
추가하려는 값이 인덱스 앞에 있으면 모든 값을 뒤로 한칸씩 밀어야한다
추가하려는 값이 인덱스 뒤쪽에 있으면 그 값 뒤로만 뒤로 한칸씩 밀어야한다
만약에 요소가 100개가 있는 배열에 어떤 요소를 맨 앞에 추가한다면
연산에 걸리는 단계수 : 추가 1번 + 요소 뒤로 미루기 100번 = 101번
만약에 요소가 100개가 있는 배열에 어떤 요소를 맨 뒤에 추가한다면
연산에 걸리는 단계수 : 추가 1번
(4) 삭제 : O(N)
arr[] = { 김, 신, 이 , 박, 원 , 윤}
arr [0] | arr[1] | arr[2] | arr[3] | arr[4] | arr[5] | |
값 | 김 | 신 | 이 | 박 | 원 | 윤 |
인덱스 | 0 | 1 | 2 | 3 | 4 | 5 |
메모리 주소 | 1010 | 1011 | 1012 | 1013 | 1014 | 1015 |
(상황 1) 마지막 인덱스에 있는 윤을 삭제한다
컴퓨터는 메모리 주소 1015에 있는 "윤" 값을 삭제한다
(상황 2) 처음이나 중간에 있는 인덱스2 위치에 "이"를 삭제한다
arr [0] | arr[1] | arr[2] | arr[3] | arr[4] | arr[5] | |
값 | 김 | 신 | 박 | 원 | 윤 | |
인덱스 | 0 | 1 | 2 | 3 | 4 | 5 |
메모리 주소 | 1010 | 1011 | 1012 | 1013 | 1014 | 1015 |
박, 원, 윤 값을 앞으로 한칸씩 땡겨야한다
"박" 을 인덱스 2으로 바꾸고 , 메모리 주소도 1012으로 바꾼다
"원" 을 인덱스 3으로 바꾸고 , 메모리 주소도 1013으로 바꾼다
"윤" 을 인덱스 4으로 바꾸고 , 메모리 주소도 1015으로 바꾼다
연산이 4번 발생했다
삭제하려는 값이 인덱스 앞에 있으면 모든 값을 앞으로 한칸씩 땡겨줘야한다
삭제하려는 값이 인덱스 뒤쪽에 있으면 그 값까지만 앞으로 한칸씩 당겨줘야한다
만약에 요소가 100개가 있는 배열에 맨 앞 요소를 삭제한다면
연산에 걸리는 단계수 : 삭제 1번 + 요소 앞으로 당기기 99번 = 100번
만약에 요소가 100개가 있는 배열에 맨 뒤 요소를 삭제한다면
연산에 걸리는 단계수 : 삭제 1번
[5] 배열 메서드
(1) 복사하기 copyOf()
1. 배열의 일부를 복사해서 새로운 배열을 만든다
int [] new_arr = Arrays.copyOfRange(arr,인덱스 0,인덱스 5)
2. 배열 전체를 복사하여 새로운 배열을 만든다
int[] new_arr = Arrays.copyOf(arr, arr.length);
3. List 배열을 String 배열로 복사한다
String [] new_arr =Arrays.copyOf(arr,Arrays.asList(arr).size());
4. String 배열을 List 배열로 복사한다
String[] arr = {"java", "hello", "hi"};
List<String> str_List = Arrays.asList(arr);
(2) 비교하기 .equals()
1. 비교하기
if(arr[0].equals(arr[1])) {
return true;
}
2. 2차원 배열 비교하기
String[][] arr1 = new String[][] {
{"ja" ,"va"},
{"Hel","lo"}
}
String[][] arr2 = new String[][] {
{"ja" ,"va"},
{"Hel","lo"}
}
String str = Arrays.deepEquals(arr1,arr2) //true
== 는 주소값을 비교한다
(3) 문자열 List 를 문자열 배열로 바꾼다
List<String> list = Arrays.asList("java", "hello hi");
(1) String[] arr = list.toArray(new String[0]);
(2) String[] arr = list.stream().toArray(String[]::new);
(3) String[] arr = Arrays.copyOf(list.toArray(), list.size(), String[].class);
(4) String[] array = new String[list.size()];
System.arraycopy(list.toArray(), 0, array, 0, list.size());
return arr = [java , hello hi]
(4) 정렬한다 sort()
1. 오름차순
Arrays.sort(arr);
Arrays.sort(arr, 인덱스1, 인덱스3);
2. 내림차순
Arrays.sort(arr, Collections.reverseOrder());
Arrays.sort(arr, 인덱스0, 인덱스5, Collections.reverseOrder());
(5) 배열 출력하기 toString()
1. int 배열 출력하기
int [] arr = { 1,2,3 }
System.out.println(Arrays.toString(arr); // [1,2,3] 출력
System.out.println(arr) // [I@14256bb 주소 출력
2. char 배열 출력하기
char [] arr = {a,b,c}
System.out.println(arr) // abc 출력
System.out.println(Arrays.toString(arr)); //[a,b,c] 출력
2-1. 굳이 문자열로 만들어서 출력하기
String str = String.copyValueOf(arr);
String str = String.valueOf(arr);
System.out.println(str);
3. String 배열 출력하기
String[] arr = {"java", "hello", "hi"};
Sring str = Arrays.toString(arr));
System.out.println(str); // [java, hello, hi] 출력
System.out.println(arr); // [Ljava.lang.String;@aec6354 주소
4. 2차원 배열 출력하기
int [][] arr = {
{1,2},
{3,4}
}
String str = Arrays.deepToString(arr);
System.out.println(str); // [ [1,2],[3,4] ] 출력
5. String 문자열을 배열로 변환하기
String str = "Hello World";
char[] arr = str.toCharArray();
System.out.println(Arrays.toString(arr)); //[H, e, l, l, o, , W, o, r, l, d] 출력
(6) 랜덤 넣기 섞기
1. 배열의 길이만큼 돌면서 값을 넣는다 1,2,3,4,5...
for(int i=0; i <arr.length; i++) {
arr[i]= i+1;
}
2. 배열의 길이만큼 돌면서 0~10 랜덤값을 넣는다
for(int i=0; i <arr.length; i++) {
arr[i]= (int) (Math.random()*10) +1;
}
3. 0~9 랜덤값을 n변수에 넣은뒤 임의의 tmp 변수에 넣어서 인덱스 이동을 시켜서 값을 이동시킨다
1,2,3,4,5 ...-> 3,2,5,4...
for(int i=0; i<100; i++) {
int n= (int)(Math.random() *10);
int tmp =Arr[0];
arr[0] =arr[n]
arr[n] =tmp;
}
4. 배열1의 값을 랜덤으로 섞어서 새로운 배열을 복사한다
int[] arr1= {1,2,3,4,5,6,7,8,9};
int[] arr2 = new int[3];
for(int i=0; i < arr1.length;i++) {
int j = (int)(Math.random() * arr1.length);
int tmp =0;
tmp = arr1[i];
arr1[i] = arr1[j];
arr1[j] =tmp;
}
System.arraycopy(arr1, 0, arr2, 0, 3);
5. 배열1의 각각의 단어를 알파벳을 조각내어 섞어서 정답 맞추기
String[] words = {"television", "computer", "mouse", "phone"};
Scanner scanner = new Scanner(System.in);
for(int i= 0; i <words.length;i++) { //4
char[] question = words[i].toCharArray();
for(int j=0; j < question.length;j++) {
int r =(int)(Math.random() * question.length);
char tmp = question[j];
question[j] = question[r];
question[r] = tmp;
}
(7) 배열을 이용하여 알파벳 출력하기
public class CharArrayTest {
public static void main(String[] args) {
char[] alpahbets = new char[26];
char ch = 'A';
for(int i = 0; i<alpahbets.length; i++) {
alpahbets[i] = ch++;
}
for(char alpha : alpahbets) {
System.out.println(alpha +","+ (int)alpha);
}
}
}
[6] 배열의 값 추가 , 삭제 예시
배열 클래스
package ch03;
public class MyArray {
int[] intArr; // 배열 선언
int count; // 요소 갯수
public int ARRAY_SIZE;
public static final int ERROR_NUM = -999999999;
public MyArray()
{
count = 0;
ARRAY_SIZE = 10; //배열크
intArr = new int[ARRAY_SIZE];
}
public MyArray(int size)
{
count = 0;
ARRAY_SIZE = size;
intArr = new int[size];
}
public void addElement(int num)
{
if(count >= ARRAY_SIZE){
System.out.println("not enough memory");
return;
}
intArr[count++] = num;
}
// 요소를 추가하려고할때 마지막 인덱스위치에 있는 값이 한칸씩 이동해야한다
public void insertElement(int position, int num)
{
int i;
if(count >= ARRAY_SIZE){ //한칸씩 이동이 못할정도로 꽉 찬 경우
System.out.println("not enough memory");
return;
}
if(position < 0 || position > count ){ //index error
System.out.println("insert Error");
return;
}
for( i = count-1; i >= position ; i--){
intArr[i+1] = intArr[i]; // 하나씩 이동
}
intArr[position] = num;
count++;
}
//요소 삭제하기
public int removeElement(int position)
{
int ret = ERROR_NUM;
if( isEmpty() ){
System.out.println("There is no element");
return ret;
}
if(position < 0 || position >= count-1 ){ //index error
System.out.println("remove Error");
return ret;
}
ret = intArr[position];
for(int i = position; i<count -1; i++ )
{
intArr[i] = intArr[i+1];
}
count--;
return ret;
}
public int getSize()
{
return count;
}
public boolean isEmpty()
{
if(count == 0){
return true;
}
else return false;
}
public int getElement(int position)
{
if(position < 0 || position > count-1){
System.out.println("검색 위치 오류. 현재 리스트의 개수는 " + count +"개 입니다.");
return ERROR_NUM;
}
return intArr[position];
}
public void printAll()
{
if(count == 0){
System.out.println("출력할 내용이 없습니다.");
return;
}
for(int i=0; i<count; i++){
System.out.println(intArr[i]);
}
}
public void removeAll()
{
for(int i=0; i<count; i++){
intArr[i] = 0;
}
count = 0;
}
}
package ch03;
public class MyObjectArray {
private int cout;
private Object[] array;
public int ARRAY_SIZE;
public MyObjectArray()
{
ARRAY_SIZE = 10;
array = new Object[ARRAY_SIZE];
}
public MyObjectArray(int size)
{
ARRAY_SIZE = size;
array = new Object[ARRAY_SIZE];
}
}
package ch03;
public class MyArrayTest {
public static void main(String[] args) {
MyArray array = new MyArray();
array.addElement(10);
array.addElement(20);
array.addElement(30);
array.insertElement(1, 50);
array.printAll();
System.out.println("===============");
array.removeElement(1);
array.printAll();
System.out.println("===============");
array.addElement(70);
array.printAll();
System.out.println("===============");
array.removeElement(1);
array.printAll();
System.out.println("===============");
System.out.println(array.getElement(2));
}
}
'🌈 백엔드 > 자료구조' 카테고리의 다른 글
자료구조_선형구조 ③ 스택 (0) | 2023.03.08 |
---|---|
자료구조_선형구조 ②연결리스트 LinkedList (2) | 2023.02.16 |
자료구조_선형구조 ① 연접리스트 : 행렬 (0) | 2023.02.14 |
자료구조_선형구조 ① 연접리스트 : 배열리스트 ArrayList (0) | 2023.02.11 |
자료구조_ 자료구조 & 알고리즘 (0) | 2023.02.11 |
댓글